When evaluating Android applications, I often use dex2jar to take an Android APK file and convert it to a Java JAR file. With the APK-turned-JAR file I can examine the decompiled Java source for the application using JD-GUI or Mike Strobel’s Procyon.
Procyon is the far superior Java decompilation tool, which gracefully handles many conditions that JD-GUI cannot. Still, Procyon requires a few additional steps to use as a command-line tool, while Procyon has a nice GUI interface for quick and easy analysis (to be fair, Procyon does have a third-party GUI interface as well, though it lacks some of the features in JD-GUI).
As part of an exercise I am writing for my SANS Institute SEC575: Mobile Device Security and Ethical Hacking course, I needed to force the student’s hand and require them to use Procyon. I needed to reproduce a situation where my sample code was not decompiled by JD-GUI properly. I lowered my standards enough to look at page 2 of Google search results, but I still could not find an example of Java code that could not be decompiled by JD-GUI.
Looking through some APK files I had handy, I spotted an method that JD-GUI could not handle. Reversing the same code with Procyon gave me the method source, which I was able to narrow down to just a few lines of Java. If you are in the position where you want to stop someone from using JD-GUI to reverse-engineer a method, insert this code:
// Add these lines to your import section import java.io.IOException; import java.io.OutputStreamWriter; // Add this code to a method that you want JD-GUI to generate an error on OutputStreamWriter request = new OutputStreamWriter(System.out); try { request.close(); } catch (IOException e) { } finally { request = null; }
The block of code opens the System.out object (the stdout reference), and then closes it. The rest is just being graceful. When decompiled with JD-GUI, the user will see this error:
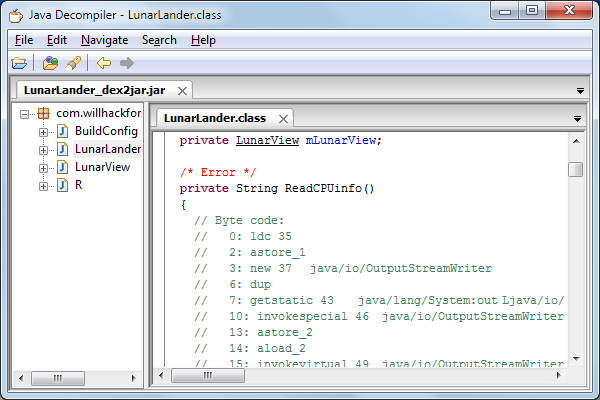
Error produced by JD-GUI when decompiling the shown code.
So, the next time you need to stop people from reversing your code, add these lines to a method, and hope that they don’t know about Procyon.
-Josh